On Saturday, February 3rd, at 1:00 AM EST (US server), we will conduct planned maintenance, and the monday.com platform will be in Read Only mode.
All mutations will be blocked during this period. Any API call containing a mutation will result in the following error: "GraphQL Action Mutations::ArchivePulse has been blocked".
The total maintenance window is estimated to last for 30 minutes. Please plan accordingly!
The new DeleteLastGroupException
error occurs when you attempt to delete or archive the only group on a board. To resolve the error, ensure that the board has more than one group.
This error will appear in API versions 2024-01
and later.

We've been updating the docs to create a more straightforward and user-friendly experience - starting with the site navigation.
The API documentation has been split into two sections as part of these updates: API reference and guides.
-
The API reference section houses docs about every object, argument, and field you can query or mutate using the API. You can now search inside the API reference docs themselves to return smaller batches of more relevant results.
-
The Guides section contains all the other important information to know when using the API like versioning, introduction to GraphQL, and error codes.
You can easily flip between the two sections (+ the changelog) using the dropdown pictured below!
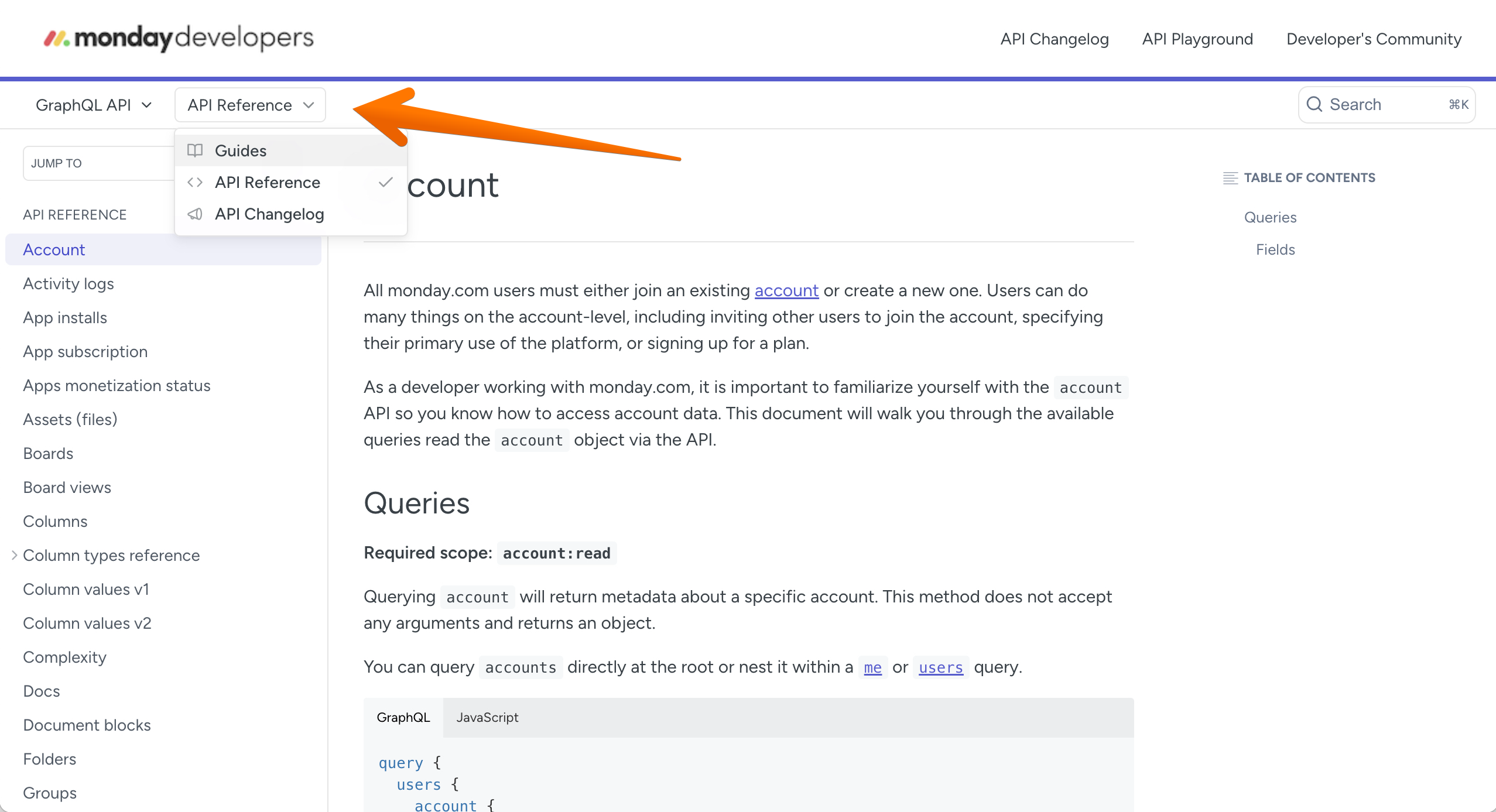
We recently added two new mutations that allow you to add or remove users from a team. These are available in API versions 2024-01
and later!
mutation {
add_users_to_team (team_id: 7654321, user_ids: [123456, 654321, 012345]) {
successful_users {
name
email
}
failed_users {
name
email
}
}
}
mutation {
remove_users_from_team (team_id: 7654321, user_ids: [123456, 654321, 012345]) {
successful_users {
name
email
}
failed_users {
name
email
}
}
}
Starting today, we have a new support form to open a technical ticket with our team! This new form helps us to route your requests and gather useful information about the issues you are experiencing. This will allow us to address your concerns more effectively and provide you with a faster resolution.
The [email protected] email address will no longer be valid. It is set to auto-close and will become a no-reply email address. Moving forward, we encourage all developers to utilize our new support form. App users should reach out via the monday.com support center as usual.
This transition is aimed at improving the support process and enhancing your overall developer experience with our API and app marketplace!
On Jan 16th 2024, we will begin a gradual release of API version 2024-01 as the stable and default version.
If you've migrated your apps already (to 2023-10
or beyond) and want to use an up-to-date API version, you can:
- Pass the version header in every request:
"API-Version" : "2024-01"
- Use the
setApiVersion
option if using the Javascript SDK
Take note of this slight update from the previous announcement – we originally communicated the change would be in effect at 00:00 GMT.
You can now query a workspace's team owners using the team_owners_subscribers
field on a workspaces
query. This field is available in API versions 2024-01
and later. You can read more about workspaces and the supported fields in our documentation!
Example
The following query would return the name and ID of the team owners in workspace 1234567.
query {
workspaces (ids: 1234567) {
team_owners_subscribers {
name
id
}
}
In API versions 2024-01
and later, you can use the new delete_teams_from_board
mutation to remove teams from a board. You can find more info about this new query in our documentation!
Example
The following example would remove teams 123456, 654321, and 012345 from board 1234567890.
query {
delete_teams_from_board (board_id: 1234567890, team_ids: [123456, 654321, 012345]) {
id
}
}