In API version 2024-01
, we fixed a typo in the UserUnauthorizedException
error. The error code used to read "UserUnauthroizedException", but it now reads "UserUnauthorizedException".
After
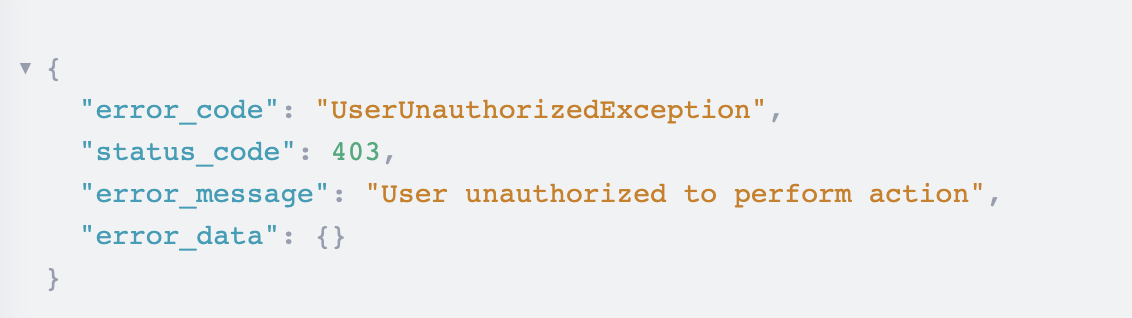
Before
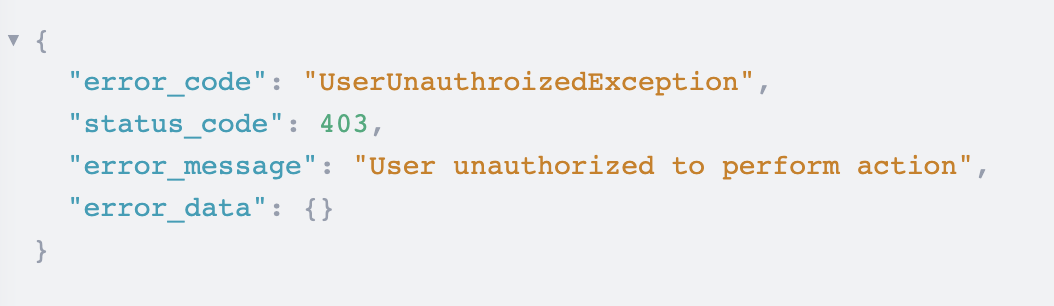
In API version 2024-01
, we fixed a typo in the UserUnauthorizedException
error. The error code used to read "UserUnauthroizedException", but it now reads "UserUnauthorizedException".
After
Before
We recently added the ability to sort and filter items_page
results by either their last updated or creation dates.
You can do so by entering either "__creation_log__"
or "__last_updated__"
as the column ID in the order_by
field of your query. The items will be returned chronologically, with the oldest listed first.
Please note that you are not required to have a last updated or creation log column on your board to use this sorting mechanism.
The following example would return the first 50 items on board 1234567890 with a blank status column value, and they would be listed chronologically (oldest to newest) according to their creation date.
query {
boards (ids: 1234567890){
items_page (limit: 50, query_params: {order_by: [{column_id: "__creation_log__"}], rules: [{column_id: "status", compare_value: [5]}], operator: and}) {
cursor
items {
id
name
}
}
}
}
We've recently made a few updates and improvements to our webhooks to help provide you with a better developer experience. Let's check them out!
app_webhooks_only
argumentThis new argument returns just the webhooks created by the app initiating the request when querying the webhooks
object through the API.
query {
webhooks (app_webhooks_only:true, board_id:1234567890) {
id
event
config
}
}
Before these updates, we were missing a feature that sent the authorization header for some webhooks. We added the missing feature, so now the authorization header will be included in all webhooks. Make sure to start validating your requests from monday!
Please note that this only applies to apps created in the Developer Center. You will not receive the authorization header if your app uses a personal token.
While webhooks created by apps were always labeled internally on monday as belonging to a specific app, we enabled this labeling in the Active integrations page on the board so users can’t remove your webhooks from the board!
In the 2023-10
API release, we introduced the items_page
object which utilizes cursor-based pagination to retrieve smaller groups of items from a large data set. This object must be nested inside of a boards
query, so it has a high complexity cost.
To help reduce that cost, we created the next_items_page
object which can be used at the root of your query to paginate through the data set. It takes both the cursor
and limit
arguments and retrieves the following page of items in the data set.
Now let's break it down a bit...
Say you only want to retrieve items with a blank status column from board 1234567890. This board contains thousands of items that cannot be retrieved simultaneously, so you can use cursor-based pagination.
The query below would return the ID and name of the first 50 items with a blank status column and a cursor value that represents the position in the data set after returning 50 items.
query {
boards (ids:1234567890) {
items_page (limit: 50, query_params: {rules: {column_id: "status", compare_value: [5], operator:any_of}}) {
cursor
items {
id
name
}
}
}
}
You can then use the next_items_page
object with the cursor argument from the first query to return the following 50 relevant items in the data set. After returning the next cursor value, you can continue paginating through the entire data set.
query {
next_items_page (limit: 50, cursor: "MSw5NzI4MDA5MDAsaV9YcmxJb0p1VEdYc1VWeGlxeF9kLDg4MiwzNXw0MTQ1NzU1MTE5") {
cursor
items {
id
name
}
}
}
We have removed support for the text
field on MirrorValue
, DependencyValue
, and BoardRelationValue
column values. We have replaced it with the new display_value
field to return text values for the connect boards, dependency, and mirror columns.
As part of the 2023-10 release, we created the text
field on column_values
to return the text values for each column. These calls have a high cost for the mirror, dependency, and connect board columns.
Therefore, we stopped supporting the text
field and created the display_value
field on these three column values only. These updates will help reduce the load by only returning the data when users actually need it.
The following example would return the mirror column display values (i.e., text values) on items 1234567890 and 9876543210.
query {
items (ids:[1234567890, 9876543210]) {
column_values {
... on MirrorValue {
display_value
}
}
}
}
We recently updated the DependencyValue
implementation, so you can now return both the linked_items
and the linked_item_ids
for dependency columns when querying through column_values
V2.
Please note that the DependencyValue
implementation is only available in version 2023-10
.
query {
items (ids:[1234567890, 9876543210]) {
column_values {
... on DependencyValue {
linked_item_ids
linked_items
}
}
}
}
The new after_column_id
argument on the create_column
mutation allows you to specify which column to create the new one after. This argument is available in API versions 2023-07
and later. Check out our documentation for more info!
In the following code sample, the new status column would be created after the country column.
mutation{
create_column(board_id: 1234567890, title:"Work Status", description: "This is my work status column", column_type:status, after_column_id: "country") {
id
title
description
}
}
The deprecated items
field on the groups
object will be removed and replaced with items_page
in API version 2023-10
. Please see our previous announcement for more information about the items_page
field.
Due to the new year's holiday, we are delaying the API versioning release dates – the last day you have to migrate your apps is now January 15th, 2024.
Thereofre, the following will happen on January 16th at 9:00 AM GMT:
2024-01
will be released for preview2023-07
will be deprecated2023-07
as the default version when sending a request without a header