Making your first request
Setting up a monday.com account
The first step is to sign up and create a trial account. You can also create a developer account strictly for developing apps. If you already have a monday.com account, skip to the next step!
Enable Developer mode
Developer mode is a valuable tool that makes developing with monday easier by exposing template IDs, column IDs, doc IDs, and more. It allows you to easily retrieve these IDs, which many methods in our API require. Follow these steps to enable it:
- Click on your profile picture in the top right corner of your monday account.
- Select monday.labs.
- Type Developer mode in the search bar.
- Click Activate and close the modal.
- Wait for the page to refresh.
- Refresh the page and reopen your profile menu.
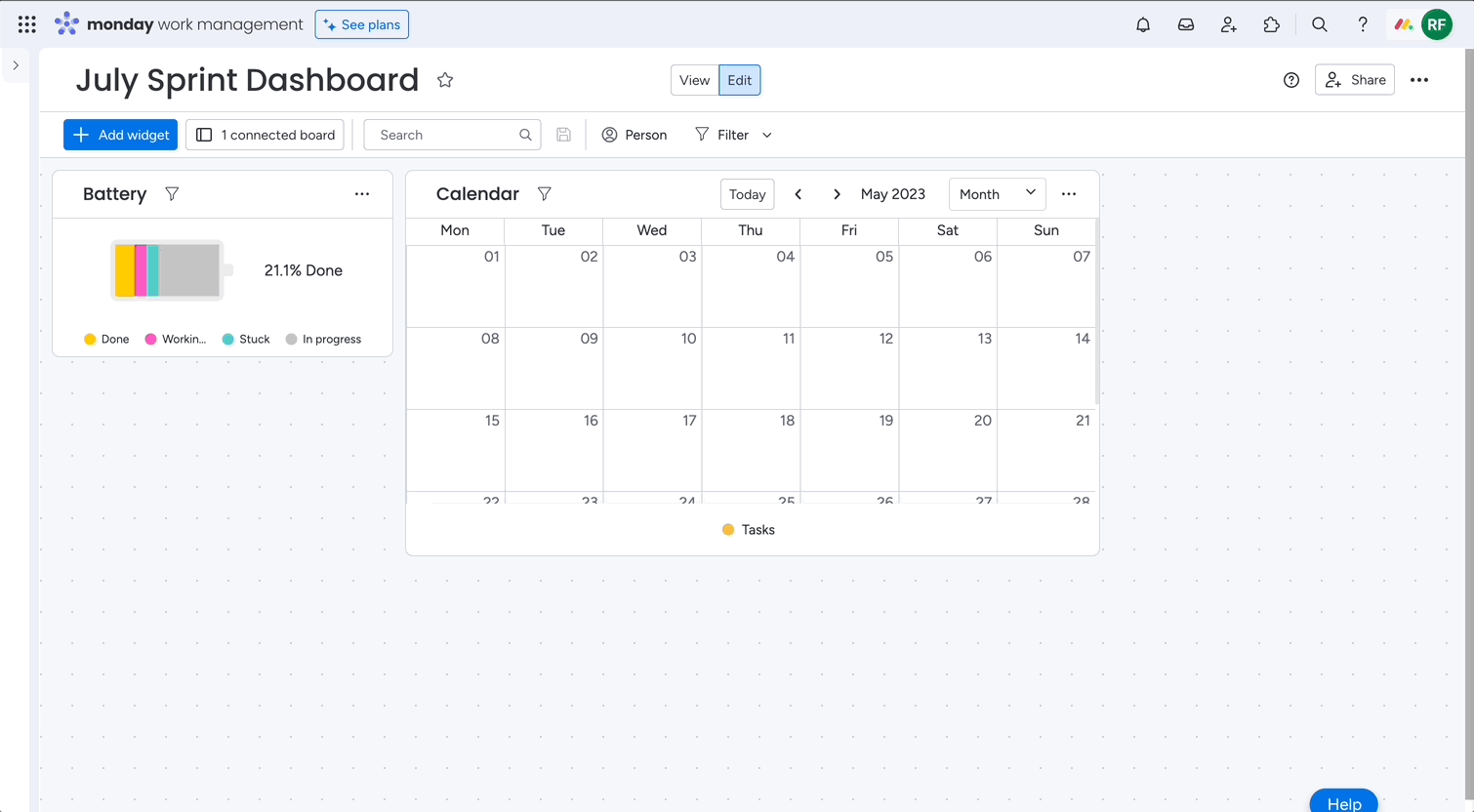
Authenticating with the API
After setting up your monday.com account, you must authenticate with an Access Token. To learn about how your apps should authenticate with our API, check out our authentication documentation.
Using the API
After authenticating your app, you're ready to start using the API! If you’re new to monday.com, it’s best to start by learning the basics of the platform in our Help Center.
If you are already familiar with the monday.com building blocks, you can explore our GraphQL schema here or in our API playground.
GraphQL APIs use a single endpoint for all operations (unlike REST APIs). Our API endpoint is:
https://api.monday.com/v2
Requests to the API should follow these rules:
- POST request with a JSON-formatted body
- Access token must be sent in the
Authorization
header. Learn more here: Authentication - All queries (including mutations) should be sent with the
query
key in your JSON body - Optional variables should use the
variables
key Content-Type
header must beapplication/json
unless you're uploading FilesAPI-Version
header should be used to call a specific API version
Your JSON request body should look like this:
{
"query": "query {...}",
"variables": { "var1": "value1", "var2": "value2", ... }
}
Examples
Below are some examples of how to access the monday.com API using different languages:
curl --location 'https://api.monday.com/v2/' \
--header 'Authorization:YOUR_API_KEY_HERE' \
--header 'API-Version: 2023-07' \
--header 'Content-Type: application/json' \
--data '{"query":"query { boards (ids: 1234567890) {name}}"}'
curl -X POST -H "Content-Type:application/json" -H "Authorization:YOUR_API_KEY_HERE" -H "API-Version:2023-07" -d "{\"query\":\"query{boards(ids:1234567890){name}}\"}" "https://api.monday.com/v2/"
fetch ("https://api.monday.com/v2", {
method: 'post',
headers: {
'Content-Type': 'application/json',
'Authorization' : 'YOUR_API_KEY_HERE',
'API-Version' : '2023-04'
},
body: JSON.stringify({
'query' : 'query{boards (limit:1) {id name} }'
})
});
<?php
$token = 'YOUR_TOKEN_HERE';
$apiUrl = 'https://api.monday.com/v2';
$headers = ['Content-Type: application/json', 'Authorization: ' . $token, 'API-version' : 2023-04];
$query = 'query { boards (limit:1) {id name} }';
$data = @file_get_contents($apiUrl, false, stream_context_create([
'http' => [
'method' => 'POST',
'header' => $headers,
'content' => json_encode(['query' => $query]),
]
]));
$responseContent = json_decode($data, true);
echo json_encode($responseContent);
?>
import requests
import json
apiKey = "YOUR_API_KEY_HERE"
apiUrl = "https://api.monday.com/v2"
headers = {"Authorization" : apiKey, "API-Version" : "2023-04"}
query2 = 'query { boards (limit:1) {id name} }'
data = {'query' : query2}
r = requests.post(url=apiUrl, json=data, headers=headers)
Below is an example of what the data returned looks like. Notice that GraphQL will return data in the same structure defined in your query:
{
"data": {
"boards": [
{
"id": "12345678",
"name": "My Amazing CRM Board"
}
]
},
"account_id": 98765
}
Updated 18 days ago