Learn how to read and update webhooks using the monday.com platform API, verify webhook URLs, and retry policies
Webhooks (also called a web callback or HTTP push API) are ways to provide real-time information and updates. They deliver data to other applications as it happens, making webhooks much more efficient for both providers and consumers.
Our webhook integration provides real-time updates from monday.com boards, making it a valuable alternative to constantly polling the API for updates. You can use it to subscribe to events on your boards and get notified by an HTTP post request to a specified URL with the event information as a payload.
If you're building an app for the marketplace, check out our app lifecycle webhooks guide.
Adding a webhook to a board
Follow these steps to add a webhook to one of your boards:
- Open the Automations Center in the top right-hand corner of the board.
- Click on Integrations at the bottom of the left-pane menu.
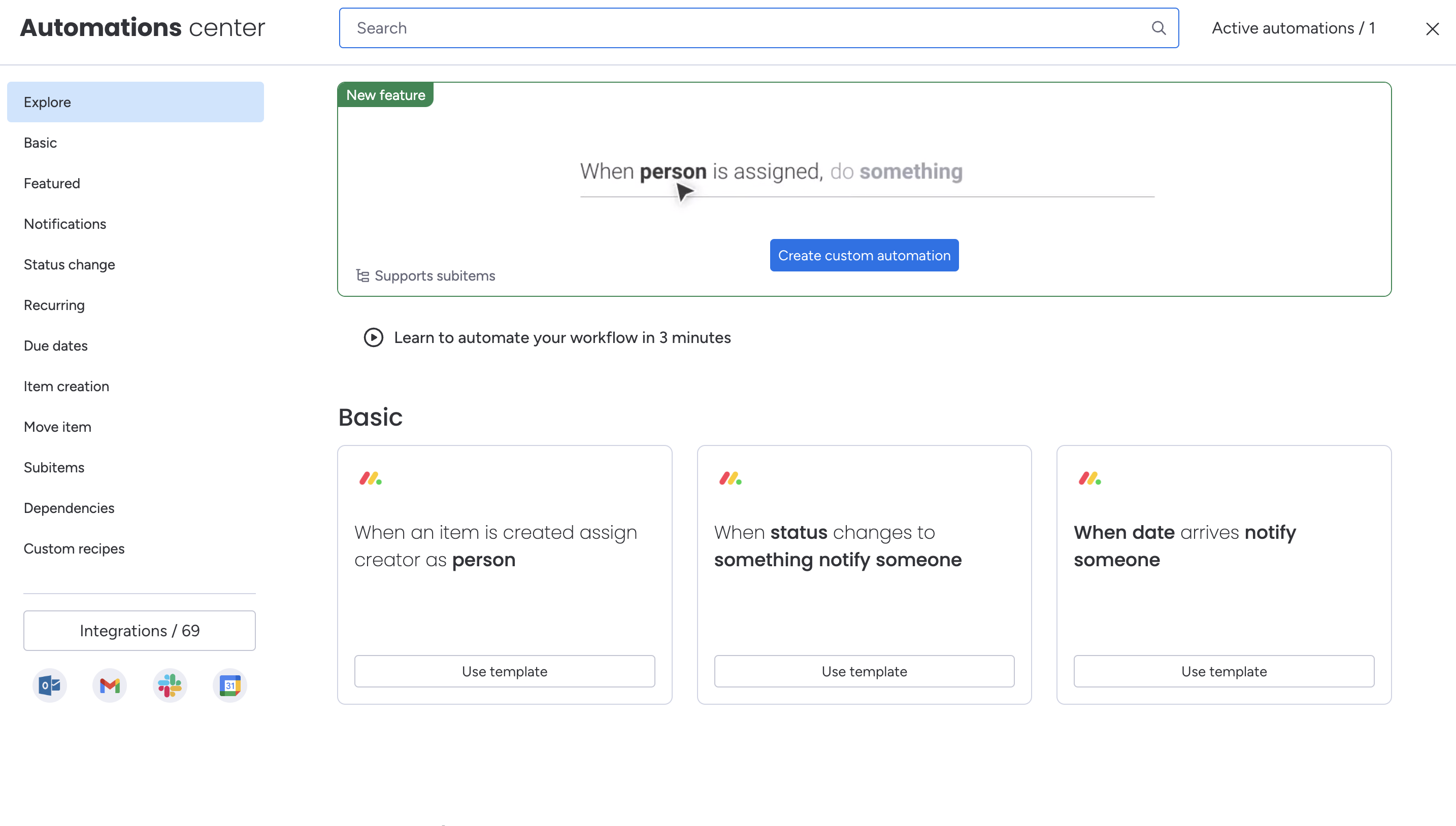
- Search for webhooks and find our webhooks app.
- Select the webhook recipe of your choosing.
- Provide the URL that will receive the event payload. Please note that our servers will send a
challenge
to that URL to verify that you control this endpoint. Check out the verifying a webhook URL section for more info!
URL Verification
Your app should control the URL you specified. Our platform checks this by sending a JSON POST body containing a randomly generated token as a challenge
field. We expect you to return the token as a challenge
field of your response JSON body to that request.
The challenge
will look something like this, and the response body should be an identical JSON POST body.
{
"challenge": "3eZbrw1aBm2rZgRNFdxV2595E9CY3gmdALWMmHkvFXO7tYXAYM8P"
}
Here's a simple example of a webhook listener that will print the output of the webhook and respond correctly to the challenge:
app.post("/", function(req, res) { console.log(JSON.stringify(req.body, 0, 2)); res.status(200).send(req.body);})
from flask import Flask, request, abort, jsonify
app = Flask(__name__)
@app.route('/webhook', methods=['POST'])
def webhook():
if request.method == 'POST':
data = request.get_json()
challenge = data['challenge']
return jsonify({'challenge': challenge})
# print(request.json)
# return 'success', 200
else:
abort(400)
if __name__ == '__main__':
app.run(debug=True)
Authenticating requests
Some webhook requests contain a JWT in the Authorization header, which can be used to check the request is legitimate. To authenticate the request, verify the JWT's signature against the app's Signing Secret, as described in our integrations documentation.
If you want to enable this feature, create the webhook with an integration app token:
- Create a monday app & add an integration feature to it
- Generate an OAuth token for this app
- Call the
create_webhook
mutation using the OAuth token
Remove ability to turn off webhook
End-users cannot disable integration webhooks. This is so the app does not get disrupted by a curious end-user toggling a webhook on and off.
To enable this feature, make sure to create the webhook with an integration app token. Instructions to do that are in the previous section.
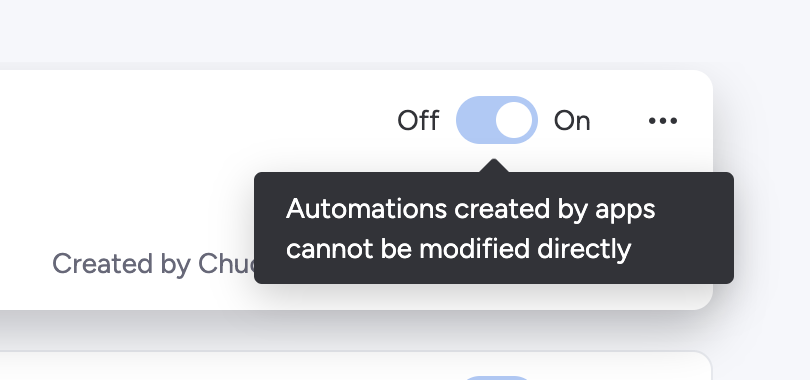
Retry policy
Requests sent through our webhook integration will retry once a minute for 30 minutes.
Queries
Required scope: webhooks:read
Querying webhooks
will return one or a collection of webhooks. This method accepts various arguments and returns an array.
You can only query webhooks
directly at the root, so it can't be nested within another query.
query {
webhooks(board_id: 1234567890){
id
event
board_id
config
}
}
let query = 'query { webhooks (board_ids: 1234567890) { id event board_id config }}';
fetch ("https://api.monday.com/v2", {
method: 'post',
headers: {
'Content-Type': 'application/json',
'Authorization' : 'YOUR_API_KEY_HERE'
},
body: JSON.stringify({
'query' : query
})
})
.then(res => res.json())
.then(res => console.log(JSON.stringify(res, null, 2)));
Arguments
You can use the following argument(s) to reduce the number of results returned in your webhooks
query.
Argument | Description |
---|---|
app_webhooks_only Boolean | Returns only the webhooks created by the app initiating the request. |
board_id ID! | The unique identifier of the board that your webhook subscribes to. |
Fields
The following fields will determine the data returned from your webhooks query. You can use the following field(s) to specify what information your webhooks
query will return.
Field | Description |
---|---|
board_id ID! | The unique identifier of the webhook's board. |
config String | Stores metadata about what specific actions will trigger the webhook. |
event WebhookEventType! | The event the webhook listens to: - change_column_value - change_status_column_value - change_subitem_column_value - change_specific_column_value - change_name - create_item - item_archived - item_deleted - item_moved_to_any_group - item_moved_to_specific_group - item_restored - create_subitem - change_subitem_name - move_subitem - subitem_archived - subitem_deleted - create_column - create_update - edit_update - delete_update - create_subitem_update |
id ID! | The webhook's unique identifier. |
Mutations
Required scope: webhooks:write
Create a webhook
The create_webhook
mutation allows you to create a new webhook via the API. You can also specify what fields to query back when you run the mutation. After the mutation runs, a webhook subscription will be created based on a specific event, so the webhook will send data to the subscribed URL every time the event happens on your board.
You can add a query param to your webhook URL if you want to differentiate between subitem and main item events. The URL must pass a verification test where we will send a JSON
POST body request containing a challenge
field. We expect your provided URL to return the token as a challenge
field in your response JSON
body to that request.
mutation {
create_webhook (board_id: 1234567890, url: "https://www.webhooks.my-webhook/test/", event: change_status_column_value, config: "{\"columnId\":\"status\", \"columnValue\":{\"$any$\":true}}") {
id
board_id
}
}
fetch ("https://api.monday.com/v2", {
method: 'post',
headers: {
'Content-Type': 'application/json',
'Authorization' : 'YOUR_API_KEY_HERE'
},
body: JSON.stringify({
query : "mutation { create_webhook (board_id: 1234567890, url: \"https://www.webhooks.my-webhook/test/\", event: change_status_column_value, config: \"columnId\":\"status\", \"columnValue\":{ {\"$any$\":true}) { id board_id } }"
})
})
Arguments
You can use the following argument(s) to define the new webhook's characteristics.
Argument | Definition |
---|---|
board_id ID! | The board's unique identifier. If creating a webhook for subitem events, send the main/parent board ID. |
config JSON | The webhook configuration. |
event WebhookEventType! | The event to listen to: - change_column_value - change_status_column_value - change_subitem_column_value - change_specific_column_value - change_name - create_item - item_archived - item_deleted - item_moved_to_any_group - item_moved_to_specific_group - item_restored - create_subitem - change_subitem_name - move_subitem - subitem_archived - subitem_deleted - create_column - create_update - edit_update - delete_update - create_subitem_update |
url String! | The webhook URL. This argument has a limit of 255 characters. |
Note: Some events also accept the config argument used to pass configuration for the event.
Events that accept the config argument | JSON | Notes |
---|---|---|
change_specific_column_value | {"columnId": "column_id"} | Using this mutation will not support subscribing to sub-item columns at this time. You can learn how to find the column ID here. |
change_status_column_value | {"columnValue": {"index": please see note*}, "columnId": "column_id"}, | Learn how to find the index and column ID here. *The structure of the index varies based on the column type and is identical to the data returned from the API. |
item_moved_to_specific_group | {"groupId": "group_id"} | You can learn how to find the group ID here. |
Delete a webhook
The delete_webhook
mutation allows you to delete a webhook via the API. You can also specify what fields to query back when you run the mutation. After the mutation runs, it will no longer report events to the URL given.
mutation {
delete_webhook (id: 12) {
id
board_id
}
}
fetch ("https://api.monday.com/v2", {
method: 'post',
headers: {
'Content-Type': 'application/json',
'Authorization' : 'YOUR_API_KEY_HERE'
},
body: JSON.stringify({
query : "mutation { delete_webhook (id: 12) { id board_id } }"
})
})
Arguments
You can use the following argument(s) to specify which webhook to delete.
Argument | Definition |
---|---|
id ID! | The webhook's unique identifier. |
Sample payload for webhook events
Every webhook sent to your endpoint will have an event
field containing the payload with the event's data. Subitem webhooks will include a similar payload for each event but will also include the parent_item_id
and subitem board ID in their payload. You can take a deeper look into the payloads using the samples below!
"event": {
"userId": 9603417,
"originalTriggerUuid": null,
"boardId": 1771812698,
"pulseId": 1772099344,
"pulseName": "Create_item webhook",
"groupId": "topics",
"groupName": "Group Title",
"groupColor": "#579bfc",
"isTopGroup": true,
"columnValues": {},
"app": "monday",
"type": "create_pulse",
"triggerTime": "2021-10-11T09:07:28.210Z",
"subscriptionId": 73759690,
"triggerUuid": "b5ed2e17c530f43668de130142445cba"
}
"event": {
"userId": 9603417,
"originalTriggerUuid": null,
"boardId": 1772135370,
"pulseId": 1772139123,
"itemId": 1772139123,
"pulseName": "sub-item",
"groupId": "topics",
"groupName": "Subitems",
"groupColor": "#579bfc",
"isTopGroup": true,
"columnValues": {},
"app": "monday",
"type": "create_pulse",
"triggerTime": "2021-10-11T09:24:51.835Z",
"subscriptionId": 73761697,
"triggerUuid": "5c28578c66653a87b00a80aa4f7a6ce3",
"parentItemId": "1771812716",
"parentItemBoardId": "1771812698"
}
"event": {
"userId": 9603417,
"originalTriggerUuid": null,
"boardId": 1771812698,
"groupId": "topics",
"pulseId": 1771812728,
"pulseName": "Crate_item webhook",
"columnId": "date4",
"columnType": "date",
"columnTitle": "Date",
"value": {
"date": "2021-10-11",
"icon": null,
"time": null
},
"previousValue": null,
"changedAt": 1633943701.9457765,
"isTopGroup": true,
"app": "monday",
"type": "update_column_value",
"triggerTime": "2021-10-11T09:15:03.429Z",
"subscriptionId": 73760484,
"triggerUuid": "645fc8d8709d35718f1ae00ceded91e9"
}
"event": {
"userId": 9603417,
"originalTriggerUuid": null,
"boardId": 1771812698,
"pulseId": 1771812728,
"body": "<p>create_update webhook</p>",
"textBody": "create_update webhook",
"updateId": 1190616585,
"replyId": null,
"app": "monday",
"type": "create_update",
"triggerTime": "2021-10-11T09:18:57.368Z",
"subscriptionId": 73760983,
"triggerUuid": "6119292e27abcc571f90ea4177e94973"
}
"event": {
"userId": 9603417,
"originalTriggerUuid": null,
"boardId": 1771812698,
"groupId": "topics",
"pulseId": 1772099344,
"pulseName": "Create_item webhook",
"columnId": "status",
"columnType": "color",
"columnTitle": "Status",
"value": {
"label": {
"index": 3,
"text": "Status change wbhook",
"style": {
"color": "#0086c0",
"border": "#3DB0DF",
"var_name": "blue-links"
}
},
"post_id": null
},
"previousValue": null,
"changedAt": 1633944017.473193,
"isTopGroup": true,
"app": "monday",
"type": "update_column_value",
"triggerTime": "2021-10-11T09:20:18.022Z",
"subscriptionId": 73761176,
"triggerUuid": "504b2eb76c80f672a18f892c0f700e41"
}
Join our developer community!
We've created a community specifically for our devs where you can search through previous topics to find solutions, ask new questions, hear about new features and updates, and learn tips and tricks from other devs. Come join in on the fun! 😎