App life cycle events
Learn about app lifecycle events & webhooks.
Webhooks play an integral role in tracking your app's life cycle events, like installations, subscriptions, and usage. They provide real-time updates when:
- Your app is installed or uninstalled
- A subscription is created, changed, renewed, or canceled
- A trial is created or canceled
- A subscription renewal fails
The automated notifications help you save time and make it easier to track events inside your app. We recommend implementing them from the very beginning to start building a complete picture of your app's activities over time.
If you didn't implement them from the beginning - have no fear! You can start using webhooks at any time, but please note that you will not receive any historic webhook information.
Available webhooks
Installations
We have two webhooks to track your app's installation activity: install and uninstall.
- Install: The
install
webhook containing subscription data will be sent to the app once a user installs an app for the first time. This webhook will be sent for all subscription types, including free ones. It may also be sent for a paid subscription again if the user reinstalls an app that they previously paid for but then uninstalled. Please note thataccount_tier
oraccount_max_users
fields withnull
or0
values indicate that the monday.com account is in a trial period. - Uninstall: The
uninstall
webhook containing subscription data will be sent to the app as soon as a user uninstalls an app. This webhook will be sent for all subscription types, including free ones.
Subscriptions
We have a variety of webhooks to track your app's subscription activity. Webhooks containing subscription information are confidential and shared with you under our developer terms.
- Subscription created: The
app_subscription_created
webhook containing subscription data will be sent to the developer when a user purchases a plan. - Subscription changed: The
app_subscription_changed
webhook containing subscription data will be sent to the developer once a user upgrades or downgrades an existing, paying subscription. - Subscription renewed: The
app_subscription_renewed
webhook containing the renewed subscription information will be sent to the developer when a user's subscription renews on the renewal date. - Subscription canceled by user: The
app_subscription_cancelled_by_user
webhook containing subscription data will be sent to the developer when a user cancels their subscription. Please note that the subscription will remain active until the paid period ends. Once the renewal date passes, their subscription will not renew and the developer will receive theapp_subscription_cancelled
webhook. - Subscription canceled: The
app_subscription_cancelled
webhook containing subscription data will be sent to the developer when the subscription actually ends due to a cancellation. - Undo subscription cancellation: The
app_subscription_cancellation_revoked_by_user
webhook containing subscription data will be sent to the developer when a user undoes their subscription cancellation before the renewal date. This event signifies that the subscription will automatically renew on the renewal date. - App subscription renewal attempt failed: The
app_subscription_renewal_attempt_failed
webhook containing subscription data will be sent to the developer when the first subscription renewal attempt fails. - App subscription renewal failed: The
app_subscription_renewal_failed
webhook containing subscription data will be sent to the developer when the final subscription renewal fails. - Trial subscription started: The
app_trial_subscription_started
webhook containing subscription data will be sent to the developer when a user starts a new app trial. - Trial subscription ended: The
app_trial_subscription_ended
webhook containing subscription data will be sent to the developer when a user ends an app trial.
Requests
When an event occurs, a request is sent to your webhook URL. Each request has a JWT in the Authorization header that can be used to verify the request's legitimacy (read more here).
The request body contains the following metadata about the event itself:
{
type: "install",
data: {
app_id: 1000000000,
user_id: 2,
user_email: "[email protected]",
user_name: "User 1",
user_cluster: "other",
account_tier: "free",
account_max_users: 10000,
account_id: 777777,
account_name: "Demo Account",
account_slug: "test",
version_data: { major: 4, minor: 5, patch: 0, type: "minor" },
timestamp: "2023-06-26T00:00:00.000+00:00",
subscription: {
plan_id: "5",
renewal_date: "2023-07-10T00:00:00+00:00",
is_trial: false,
billing_period: "monthly",
days_left: 14,
pricing_version: 5
},
user_country: "IL"
}
{
type: "uninstall",
data: {
app_id: 1000000000,
user_id: 2,
user_email: "[email protected]",
user_name: "User 1",
user_cluster: "other",
account_tier: "free",
account_max_users: 10000,
account_id: 777777,
account_name: "Demo Account",
account_slug: "test",
version_data: { major: 4, minor: 5, patch: 0, type: "minor" },
timestamp: "2023-06-26T00:00:00.000+00:00",
subscription: {
plan_id: "5",
renewal_date: "2023-07-10T00:00:00+00:00",
is_trial: false,
billing_period: "monthly",
days_left: 14,
pricing_version: 5
},
user_country: "IL"
}
{
"type":"app_subscription_created",
"data":{
"app_id":1000000000,
"user_id":1,
"user_email":"[email protected]",
"user_name": "User 1",
"user_cluster": 'other',
"account_tier": 'free',
"account_name": "Demo Account",
"account_slug": "test",
"account_max_users": 10000,
"account_id":777777,
"version_data":{
"major":1,
"minor":2,
"patch":0,
"type":"minor"
},
"timestamp":"2022-06-23T00:00:00.000+00:00",
"subscription":{
"plan_id":"plan1",
"renewal_date":"2022-07-19T00:00:00+00:00",
"is_trial":false,
"billing_period":"monthly",
"days_left":26,
"pricing_version":5
}
}
}
{
"type":"app_subscription_changed",
"data":{
"app_id":1000000000,
"user_id":1,
"user_email":"[email protected]",
"user_name": "User 1",
"user_cluster": 'other',
"account_tier": 'free',
"account_name": "Demo Account",
"account_slug": "test",
"account_max_users": 10000,
"account_id":777777,
"version_data":{
"major":1,
"minor":2,
"patch":0,
"type":"minor"
},
"timestamp":"2022-06-23T00:00:00.000+00:00",
"subscription":{
"plan_id":"plan1",
"renewal_date":"2022-07-19T00:00:00+00:00",
"is_trial":false,
"billing_period":"monthly",
"days_left":26,
"pricing_version":5
}
}
}
{
"type":"app_subscription_renewed",
"data":{
"app_id":1000000000,
"user_id":1,
"user_email":"[email protected]",
"user_name": "User 1",
"user_cluster": 'other',
"account_tier": 'free',
"account_name": "Demo Account",
"account_slug": "test",
"account_max_users": 10000,
"account_id":777777,
"version_data":{
"major":1,
"minor":2,
"patch":0,
"type":"minor"
},
"timestamp":"2022-06-23T00:00:00.000+00:00",
"subscription":{
"plan_id":"plan1",
"renewal_date":"2022-07-19T00:00:00+00:00",
"is_trial":false,
"billing_period":"monthly",
"days_left":26,
"pricing_version":5
}
}
}
{
"type":"app_subscription_cancelled_by_user",
"data":{
"app_id":1000000000,
"user_id":1,
"user_email":"[email protected]",
"user_name": "User 1",
"user_cluster": 'other',
"account_tier": 'free',
"account_name": "Demo Account",
"account_slug": "test",
"account_max_users": 10000,
"account_id":777777,
"version_data":{
"major":1,
"minor":2,
"patch":0,
"type":"minor"
},
"timestamp":"2022-06-23T00:00:00.000+00:00",
"subscription":{
"plan_id":"plan1",
"renewal_date":"2022-07-19T00:00:00+00:00",
"is_trial":false,
"billing_period":"monthly",
"days_left":26,
"pricing_version":5
}
}
}
{
"type":"app_subscription_cancelled",
"data":{
"app_id":1000000000,
"user_id":1,
"user_email":"[email protected]",
"user_name": "User 1",
"user_cluster": 'other',
"account_tier": 'free',
"account_name": "Demo Account",
"account_slug": "test",
"account_max_users": 10000,
"account_id":777777,
"version_data":{
"major":1,
"minor":2,
"patch":0,
"type":"minor"
},
"timestamp":"2022-06-23T00:00:00.000+00:00",
"subscription":{
"plan_id":"plan1",
"renewal_date":"2022-07-19T00:00:00+00:00",
"is_trial":false,
"billing_period":"monthly",
"days_left":26,
"pricing_version":5
}
}
}
{
"type":"app_subscription_cancellation_revoked_by_user",
"data":{
"app_id":1000000000,
"user_id":1,
"user_email":"[email protected]",
"user_name": "User 1",
"user_cluster": 'other',
"account_tier": 'free',
"account_name": "Demo Account",
"account_slug": "test",
"account_max_users": 10000,
"account_id":777777,
"version_data":{
"major":1,
"minor":2,
"patch":0,
"type":"minor"
},
"timestamp":"2022-06-23T00:00:00.000+00:00",
"subscription":{
"plan_id":"plan1",
"renewal_date":"2022-07-19T00:00:00+00:00",
"is_trial":false,
"billing_period":"monthly",
"days_left":26,
"pricing_version":5
}
}
}
{
"type":"app_subscription_renewal_attempt_failed",
"data":{
"app_id":1000000000,
"user_id":1,
"user_email":"[email protected]",
"user_name": "User 1",
"user_cluster": 'other',
"account_tier": 'free',
"account_name": "Demo Account",
"account_slug": "test",
"account_max_users": 10000,
"account_id":777777,
"version_data":{
"major":1,
"minor":2,
"patch":0,
"type":"minor"
},
"timestamp":"2022-06-23T00:00:00.000+00:00",
"subscription":{
"plan_id":"plan1",
"renewal_date":"2022-07-19T00:00:00+00:00",
"is_trial":false,
"billing_period":"monthly",
"days_left":0,
"pricing_version":5
}
}
}
{
"type":"app_subscription_renewal_failed",
"data":{
"app_id":1000000000,
"user_id":1,
"user_email":"[email protected]",
"user_name": "User 1",
"user_cluster": 'other',
"account_tier": 'free',
"account_name": "Demo Account",
"account_slug": "test",
"account_max_users": 10000,
"account_id":777777,
"version_data":{
"major":1,
"minor":2,
"patch":0,
"type":"minor"
},
"timestamp":"2022-06-23T00:00:00.000+00:00",
"subscription":{
"plan_id":"plan1",
"renewal_date":"2022-07-19T00:00:00+00:00",
"is_trial":false,
"billing_period":"monthly",
"days_left":0,
"pricing_version":5
}
}
}
{
"type":"app_trial_subscription_started",
"data":{
"app_id":1000000000,
"user_id":1,
"user_email":"[email protected]",
"user_name": "User 1",
"user_cluster": 'other',
"account_tier": 'free',
"account_name": "Demo Account",
"account_slug": "test",
"account_max_users": 10000,
"account_id":777777,
"version_data":{
"major":1,
"minor":2,
"patch":0,
"type":"minor"
},
"timestamp":"2022-06-23T00:00:00.000+00:00",
"subscription":{
"plan_id":"plan1",
"renewal_date":"2022-07-19T00:00:00+00:00",
"is_trial":true,
"billing_period":"monthly",
"days_left":26,
"pricing_version":5
}
}
}
{
"type":"app_trial_subscription_ended",
"data":{
"app_id":1000000000,
"user_id":1,
"user_email":"[email protected]",
"user_name": "User 1",
"user_cluster": 'other',
"account_tier": 'free',
"account_name": "Demo Account",
"account_slug": "test",
"account_max_users": 10000,
"account_id":777777,
"version_data":{
"major":1,
"minor":2,
"patch":0,
"type":"minor"
},
"timestamp":"2022-06-23T00:00:00.000+00:00",
"subscription":{
"plan_id":"plan1",
"renewal_date":"2022-07-19T00:00:00+00:00",
"is_trial":true,
"billing_period":"monthly",
"days_left":26,
"pricing_version":5
}
}
}
Create a webhook
Creating webhooks for your app is a relatively straightforward process!
- Click your profile picture in the top right corner.
- Select Developers. This will open the Developer Center in another tab.
- Select the app you want to create webhooks for.
- Navigate to the Webhooks tab on the left-side menu.
- Enter your URL endpoint in the All events box. This will subscribe you to all 12 webhook events.
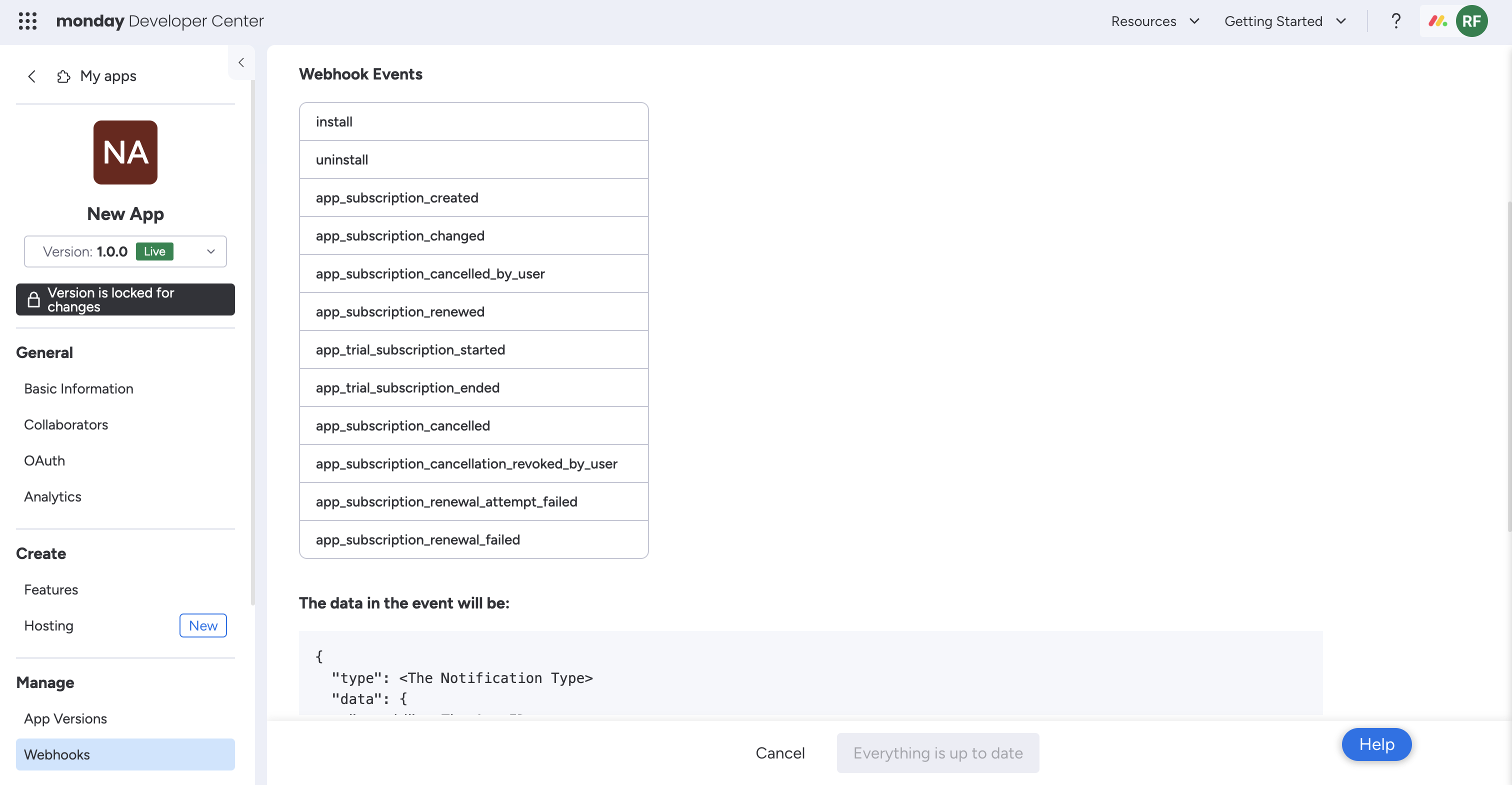
Join our developer community!
We've created a community specifically for our devs where you can search through previous topics to find solutions, ask new questions, hear about new features and updates, and learn tips and tricks from other devs. Come join in on the fun! 😎
Updated about 2 months ago